Most modern web applications nowadays consist of a frontend service hooked up to a backend API. Maintaining an API simplifies development by having a consistent place to build different kinds of applications, from web to mobile apps. It's also easier to document, version, and debug an API since it abstracts all the complexity under the hood, separating the presentation layer from the data and business logic. These boundaries can make testing your applications more effortless than doing other types of testing, whether manual or automated.
However, when testing, dealing with APIs can become a bit of a challenge at times. For instance, one issue that plagues developers and testers alike when dealing with APIs is Cross-Origin Resource Sharing, or CORS. While CORS is necessary to keep your applications secure, it creates some obstacles during your testing processes. You might run into restrictions if you don't set the proper request headers or if the testing environments have incorrect configurations. As someone who's built and tested dozens of web applications, I've dealt with enough CORS issues to last a lifetime.
Security headers and misconfigured servers aren't the only obstacles web application testers face when testing apps that communicate via APIs. Sometimes, triggering specific responses through the API is problematic, like raising an error to see how the frontend responds. Other times, you want to replicate a request with a specific body that's complex to put together through the application. In some scenarios, you might waste a lot of time waiting for developers to update the API for you to continue your testing.
Some tools like Postman can help with accessing APIs and storing the request and response cycle details, but they don't interact directly with your frontend. You can find external tools like HTTP Toolkit that help mock APIs and gather additional information for your network requests, which work well but don't integrate into the web browser. That's where Requestly can help.
What Is Requestly, and How Does It Help With Testing?
Requestly is a lightweight extension to help web developers and testers debug and test any web application directly on the browser. You can capture network traffic, modify HTTP requests, send additional headers to an API, and much more. The extension is available as a free download for most major browsers, as well as desktop apps for Windows, macOS, and Linux.
At first glance, the extension sounds similar to some of the other tools mentioned earlier, so you might think it's just a rehash of existing applications or browser extensions. However, it does provide a lot more helpful functionality that other tools don't have, and it has most of what any tester would need in a single place. There's no need to juggle multiple apps or extensions while running your tests, helping you speed up your manual testing procedures.
Here's some of the functionality provided by Requestly that helps with testing web applications:
HTTP Rules
Requestly allows you to create rules that modify the behavior of the requests and responses made from your browser. For instance, you can match a URL to change an API response so it returns the content you want or a different status code for testing. Other examples include modifying the request headers (handy for dealing with CORS issues), injecting custom JavaScript, redirecting a request to a different URL, and more.
Mock Servers
Instead of relying on a live server to do your testing, you can quickly create a mock API service with Requestly. You can set up various mock endpoints, each containing different headers, responses, and status codes. You can then use these to make requests for your testing purposes right from the browser. A mock API server can speed up development and testing cycles by simulating what you'd expect from a real API without building a full-blown backend application.
Session Replays
A recent addition to Requestly is Session Replays, which automatically records up to 5 minutes of all the user interactions you make on a page. You can then share these sessions with the rest of your team to replicate the exact flow. It goes beyond simple session recording by collecting additional data like network and console logs for the session. This functionality is a game-changer if you've ever wasted time going back and forth with bugs through screenshots or videos because of the lack of context.
Shared Lists and Team Workspaces
You can start with Requestly by creating the rules you need to help test your application. But if you work with other testers on your team, wouldn't it be nice to help them set up the same rules to ease their testing process? With Requestly, you can create a team workspace, along with a list of rules, and quickly share it with your teammates through email or the web. This feature can help your team create a repository of helpful rules to aid with collaborative development and testing.
Android App Debugger
Requestly also offers a separate library that you can integrate into your Android application codebase to obtain additional information on what's going on in your mobile app. Targeted towards Android mobile app developers, you only need to add the Requestly Android SDK as part of your app build and initialize it when loading the application. You can then quickly inspect network traffic coming in and out of the app, quickly switch API hosts without rebuilds, and more to accelerate development.
Now that we've shown a lot of the functionality provided by Requestly let's go through some examples where the browser extension can help you test your web applications faster than ever.
Setting up the Requestly browser extension
Before getting started, you'll first need to set up the Requestly browser extension. As mentioned earlier, the extension is available for Google Chrome, Mozilla Firefox, Microsoft Edge, and other browsers. The installation for all browsers is simple—download the extension for your preferred browser and open it to install it in your browser. This article won't go through the desktop application, but it should have a similar functionality to the browser extension.
Once you have the extension set up, you can click on the extension icon that should appear in the browser (typically near the address bar) to open the Requestly interface in your browser. You can also visit https://app.requestly.io/ to reach the interface.
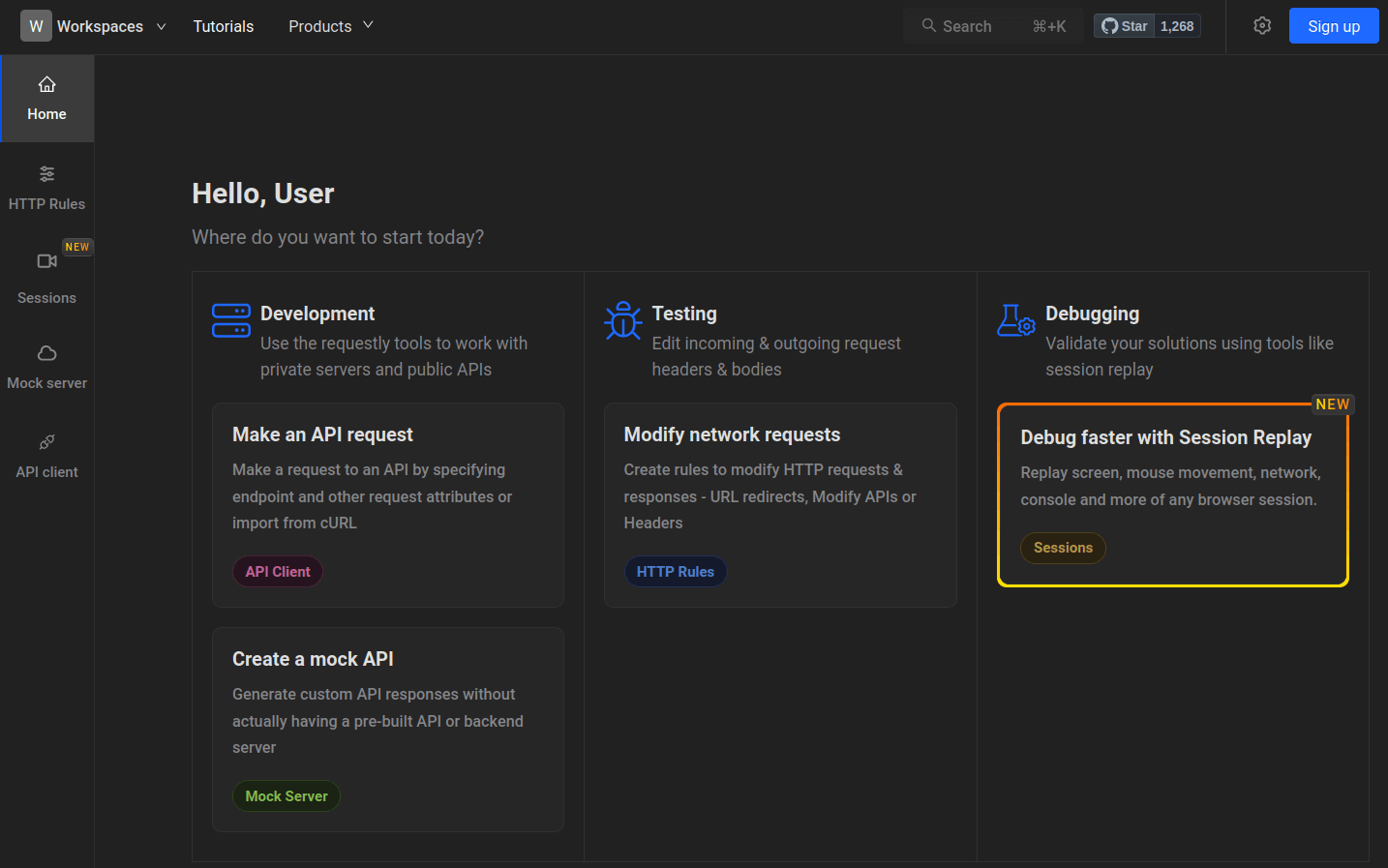
Requestly doesn't require creating an account if you plan only to use it on your own system. You will need to sign up for some functionality, like creating mock APIs. Requestly provides a free starter plan that allows you to have three active HTTP rules, set up to 10 rules, create mock servers, and more. You can remove these limits and unlock additional features like shared lists and working with GraphQL APIs with a paid subscription. The free plan is very generous to get going with Requestly, but the paid subscriptions provide excellent value for organizations and are relatively inexpensive compared to the time it can save in development and testing costs.
How to Modify Browser Requests With Requestly
When working with web applications that communicate through APIs, Requestly is extremely helpful with modifying the requests you send through the browser. This functionality makes it easy to explore how your application deals with unexpected parameters to the server without needing to change the backend services that return that data.
To show an example, we'll use an older web application I built years ago called Cash Conversion. This simple application does only one thing: it converts amounts between two currencies. The app consists of separate components: a single-page application on the frontend and a backend serving as an RESTful API that performs the currency calculations.
We can use this site to have the browser change the requests we send to the backend. Before jumping into Requestly, however, we should explore the API requests and responses to know how the application works. The simplest way to do this is by opening the web developer tools in your browser (like Chrome's DevTools or Firefox's Web Developer Tools and observing the network tab when you use the application. Here, you'll be able to see the requests and responses as they happen.
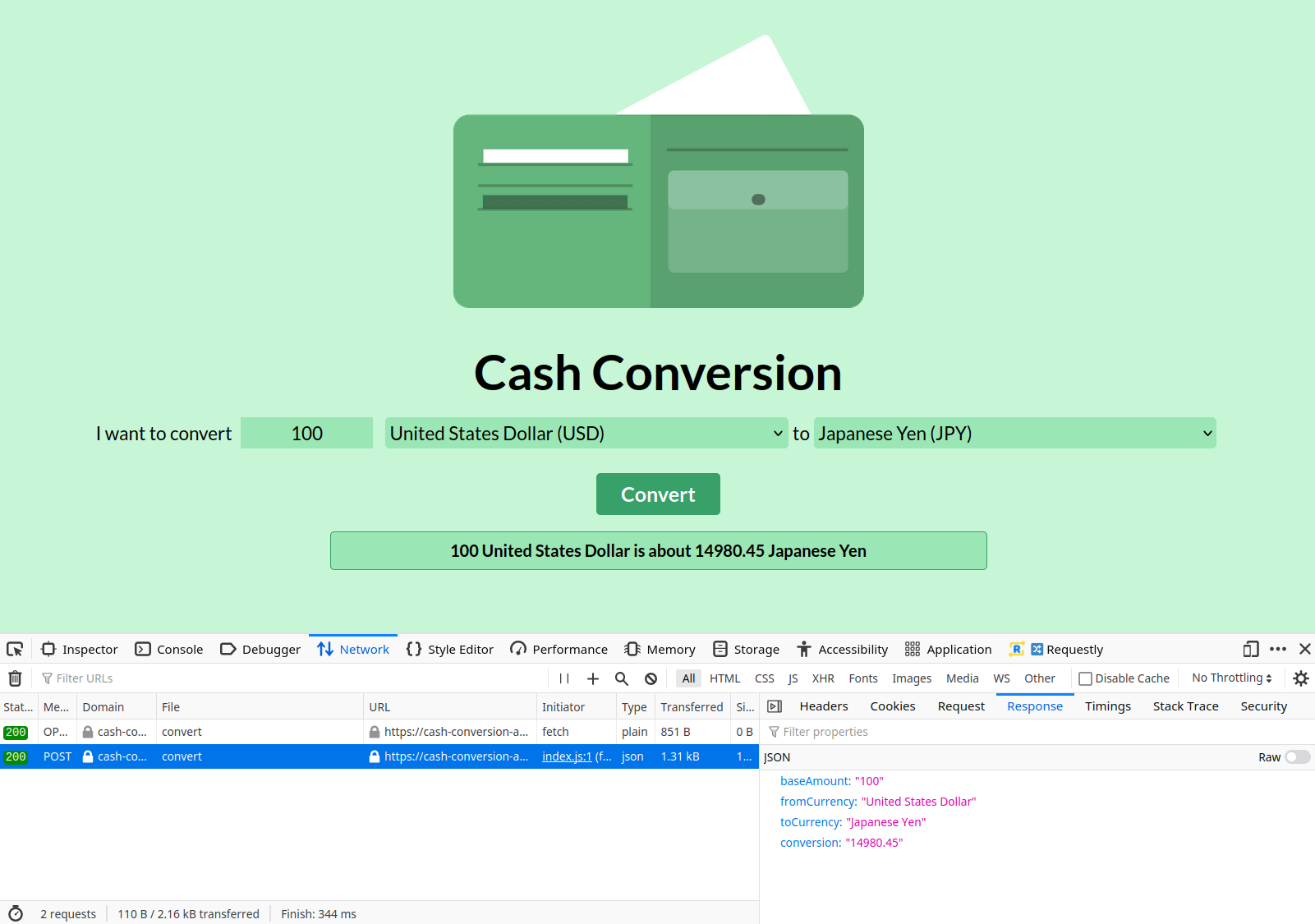
For this example, we can see the frontend made a request where it sent a few parameters in a JSON string, and the backend responded with JSON indicating that 100 U.S. Dollars is equal to approximately 14980.45 Japanese Yen, which the frontend displays on screen. With the structure of the JSON strings used for the request and response, we can use Requestly to modify them.
First, we'll explore how to modify the request body sent from the browser. Instead of using the data from the form on the page, we want to send something else. To do this, we'll open the Requestly app in the browser after installing the extension. From the Requestly starting page, go to the Modify network requests section. If you're creating a rule for the first time, you can watch a quick overview video to learn more about how this functionality works. Otherwise, you'll land on the main page to create rules for modifying requests and responses.
You'll have a few options for creating new rules, like redirecting or canceling requests, replacing parts of a URL, or managing query parameters. Since we want to modify the request body, we'll click on the Modify Request Body option and click the Create Rule button. You'll land on a new section for creating the rule to modify a request body.
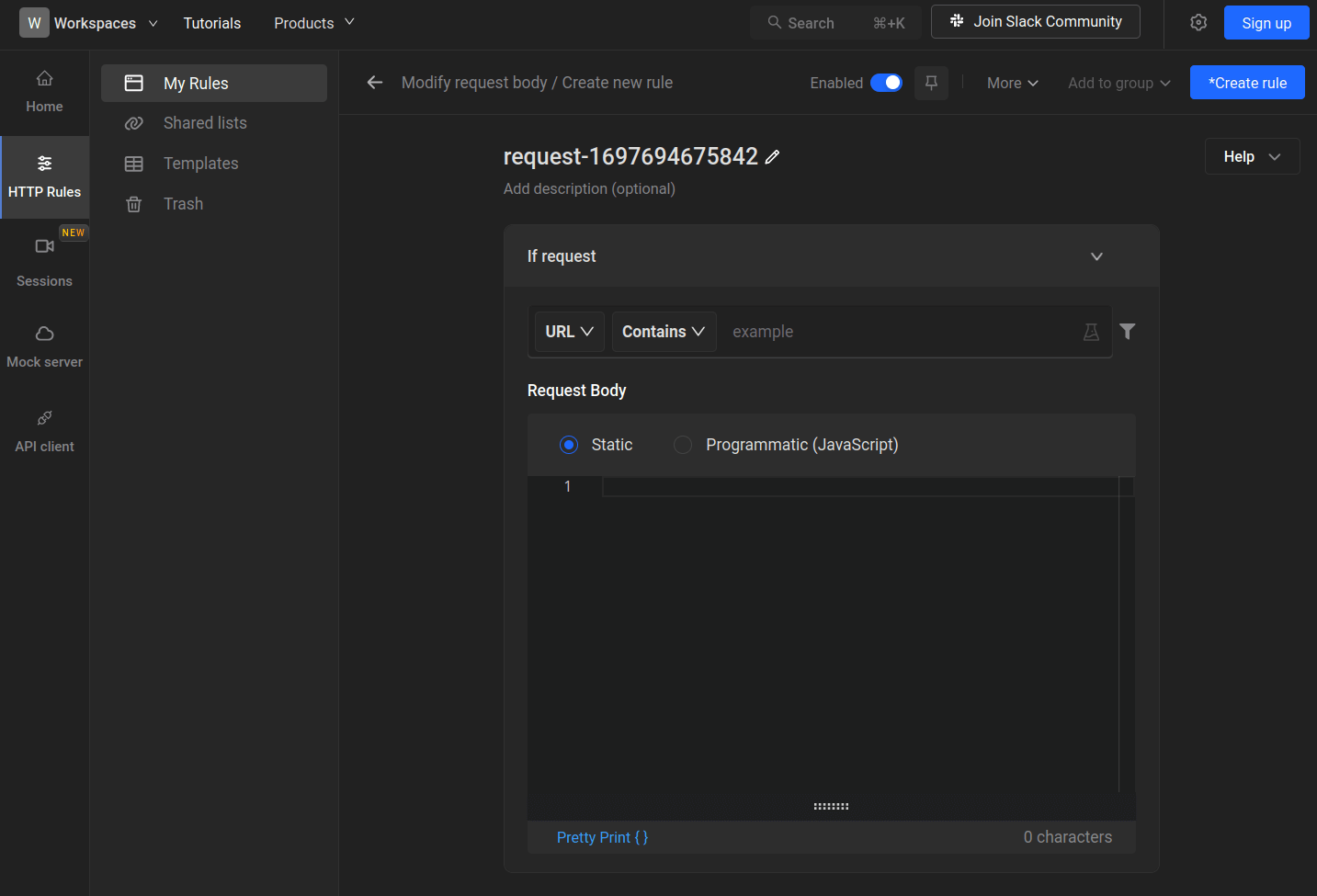
The first step for creating a rule is to specify which request you want to match. Requestly lets you match a URL, a host, or a path. You can also do exact or partial matches and use regular expressions if you want more control over what you need to compare. In this example, we'll keep it simple and match the exact API endpoint that receives the parameters for the currency conversion (in this example, it's https://cash-conversion-api.dev-tester.com/exchange_rates/convert
).
Next, you'll specify the body you want to send to that URL when making a POST
request to the endpoint. Requestly allows you to create static content, but if you want something more dynamic, you can also use a JavaScript function to set the request body. Again, we'll keep things simple by setting a static body. We'll copy the raw request body from the browser's web developer tools shown earlier and modify the amount so it always sends "1" for the currency, regardless of what's entered on the form.
Once you fill out the required details, you can rename the rule by clicking the pencil icon near the top of this section, which is helpful to keep track of all your modification rules. Ensure the Enabled switch is toggled on when finished, and click the Create rule button.
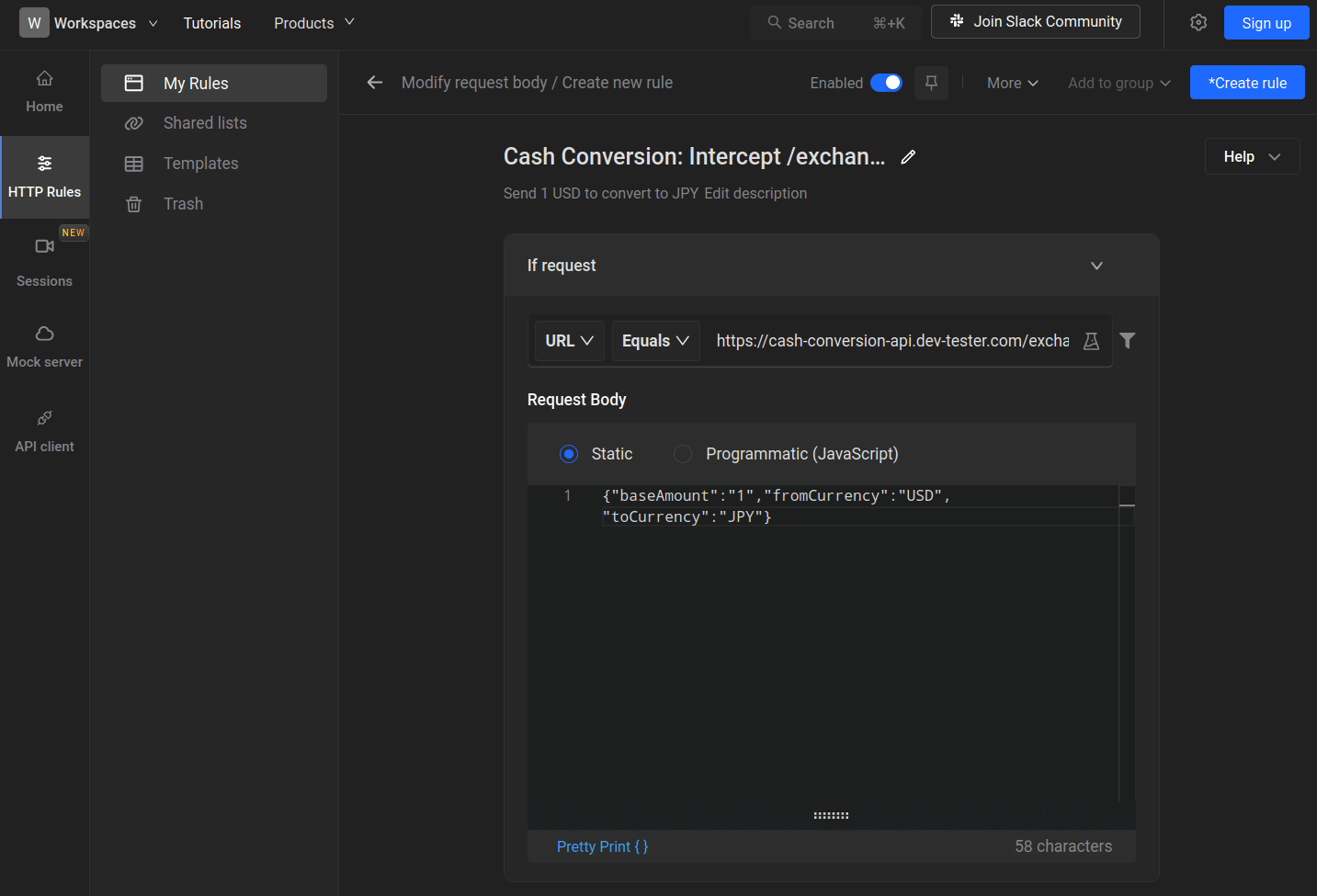
With the rule enabled on the Requestly browser extension, we can return to the Cash Conversion site and try making the same conversion as before, attempting to convert 100 U.S. Dollars to Japanese Yen. The API request to the endpoint will use the body specified in the Requestly rule, so the backend will perform the conversion using "1" instead of "100" as entered in the form.
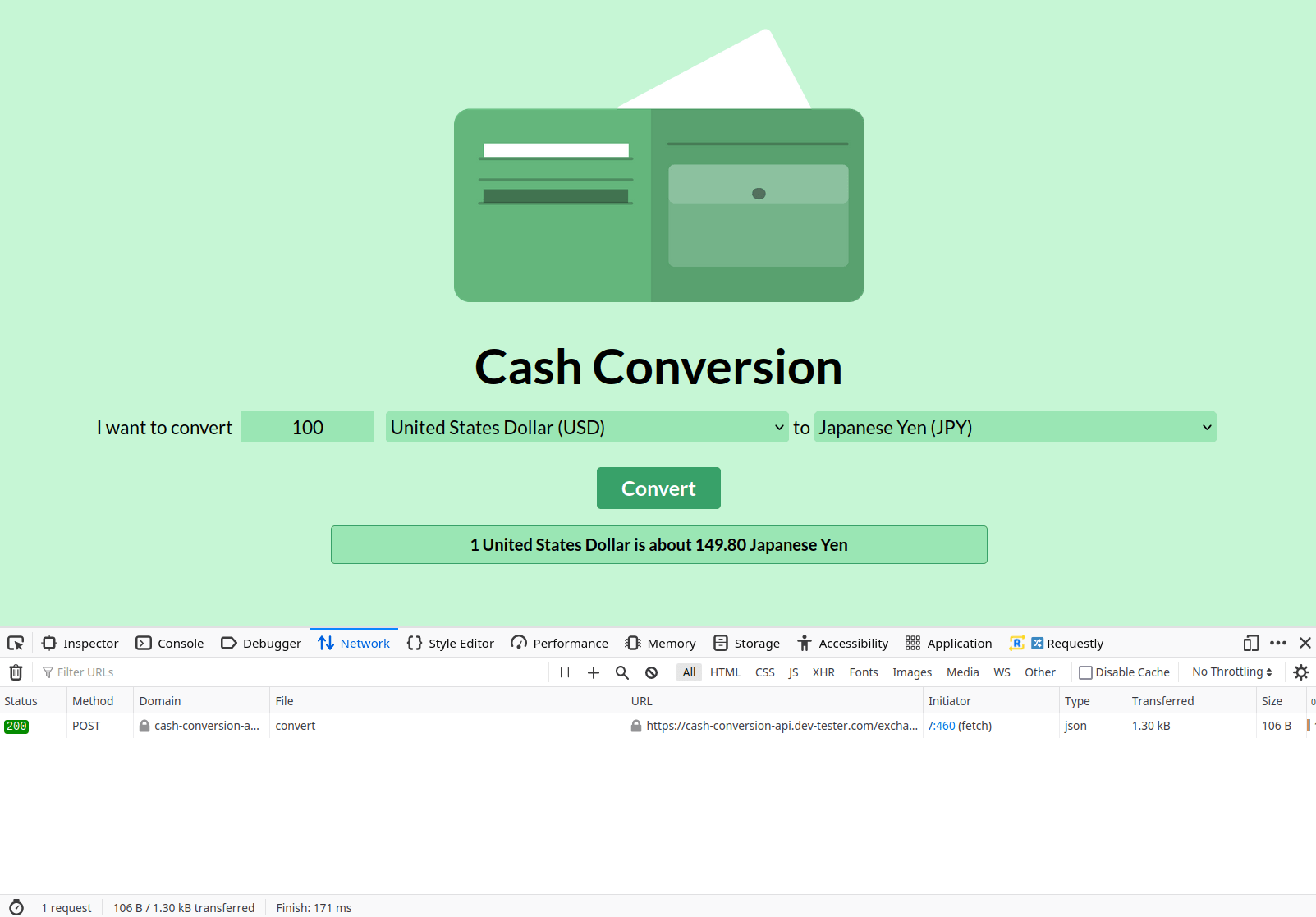
This example is somewhat trivial and not particularly useful for validating the site's functionality. However, let's see how Requestly can help us with exploratory testing. The Cash Conversion frontend has some client-side validation rules to prevent the form from submission if one of the fields is missing. Let's check out how it works.
Turn off the created Requestly rule by toggling the Enabled switch off and go back to the Cash Conversion site. This time, we'll try to submit the form by leaving the "Convert to..." field empty. When clicking the "Convert" button to submit the form, the frontend will show a message asking you to enter the amount and currencies. If you open your browser's web developer tools, you'll notice the page didn't make any requests to the backend.
The client-side validation prevents the backend from receiving invalid requests that it can't process due to missing information. However, can the backend handle missing parameters for this endpoint? In my experience, many developers rely on frontend validation and forget about handling errors on the backend. Client-side validations can fail due to a script not loading, a bug on the frontend or browser, or a malicious actor intentionally bypassing them. These oversights can lead to massive problems. The backend often returns an "Internal Server Error" response, but it can also open the door to a security breach in some cases.
We'll use Requestly to explore how the backend API responds to an invalid request body. In the Requestly rule modification screen, let's change the request body by removing the toCurrency
parameter and value in the JSON string. Re-enable the rule and save the updates to put it into effect.
Now, let's return to the Cash Conversion site and see what happens. The frontend validation is still active since we haven't changed the codebase, so we'll have to attempt a regular request. We'll fill in all the fields and submit the form as before. Requestly will send the request body with the missing parameter to the backend this time. Thankfully, the backend handles this scenario by returning an appropriate 422 Inprocessable Entity
status code, and the frontend simply indicates an error processing the conversion.
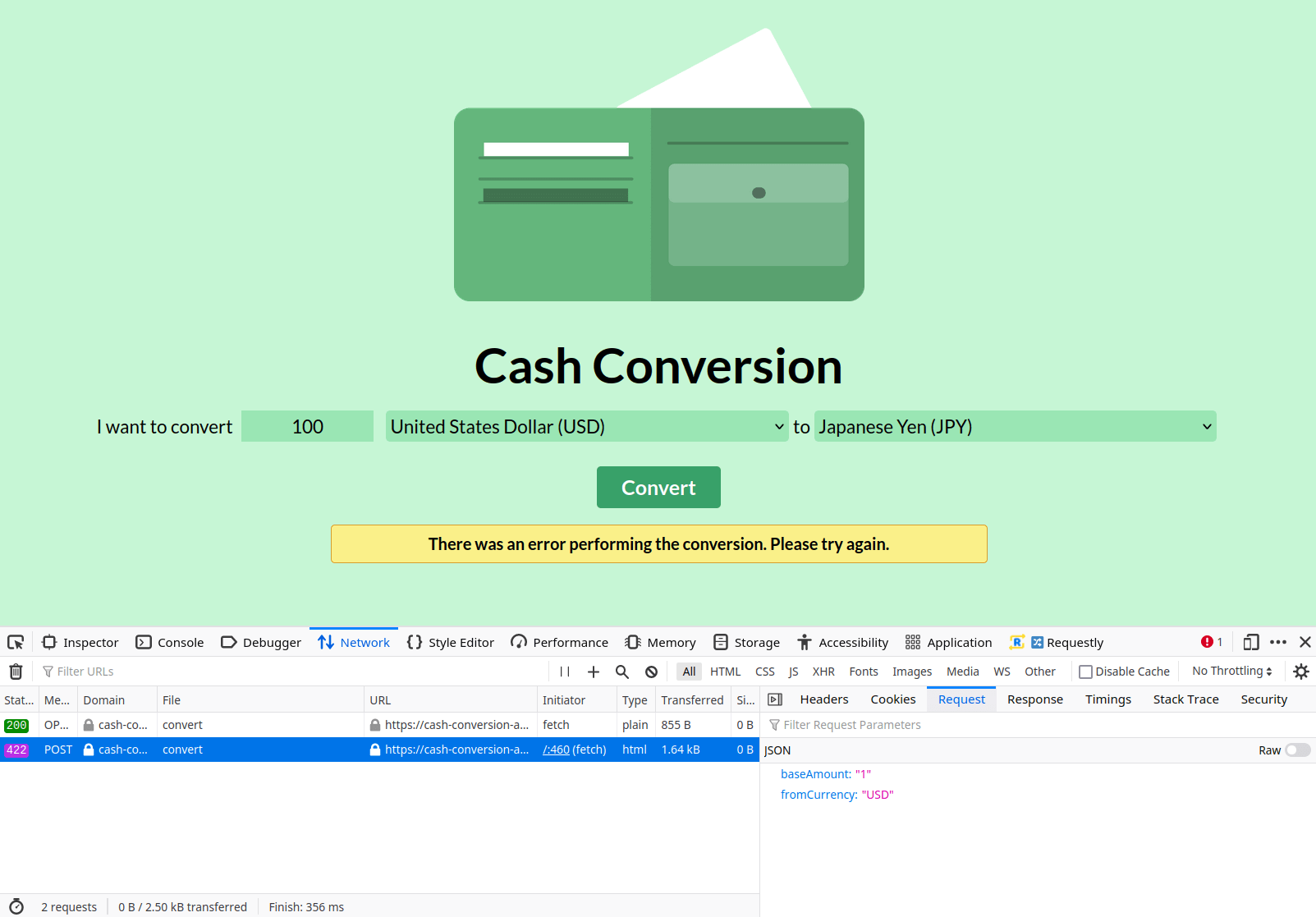
This kind of exploratory testing is incredibly valuable in software development since it can expose significant flaws due to oversights by the developers. Requestly makes it dead simple to perform these kinds of tests since we can simulate various situations and verify how the application responds.
How to Modify Browser Responses With Requestly
In the previous example, we modified the request body to verify how the backend responds to invalid requests. We can also validate how the frontend handles unexpected responses by using Requestly to change the API response from the backend. This function is helpful to quickly test edge cases and enable frontend-specific features based on a given API response, as many modern web applications do.
When we tested sending an incomplete request body to the API, we received a 422 Unprocessable Entity
response, which the Cash Conversion frontend handled well enough. But how does the application handle other scenarios, like a 429 Too Many Requests
response when a client is rate limited or a 500 Internal Server Error
response in those cases when the backend has an unhandled exception? With Requestly, we can quickly test those out.
Let's start by testing the API responding with a 429 Too Many Requests
response, which occurs when the API receives too many responses in a short period to prevent the server from getting overloaded with requests. In the Requestly app, we'll delete the previous rule to ensure it doesn't interfere with our new test. Then, we'll go to the HTTP Rules section on the sidebar and click the Modify API Response option, followed by the Create Rule button.
Similar to creating a new rule for modifying the request body, we can rename the rule and add a description to identify it later. Next, you can adjust the response of a REST API or a GraphQL API. The Cash Conversion app uses a REST API, so we'll select this option. We'll also set up the same endpoint as before so Requestly can match it when making the currency conversion on the site.
Besides being able to modify the response body similar to how we set up a custom request body previously (which we'll leave blank for this example), you have a few additional options to set. You can choose to set the response status code from the API by selecting one of the codes from the dropdown list. If left blank, the API won't modify the status code returned by the backend when accessing the matched URL. In our example, we'll set the status code to return a 429 Too Many Requests
status code.
You can also tell Requestly to bypass the request to the server entirely and immediately return the response specified in this rule. We still want to make the API request, so we'll leave this option unchecked. After filling out the required fields and enabling the rule, click the Create rule button to activate it.
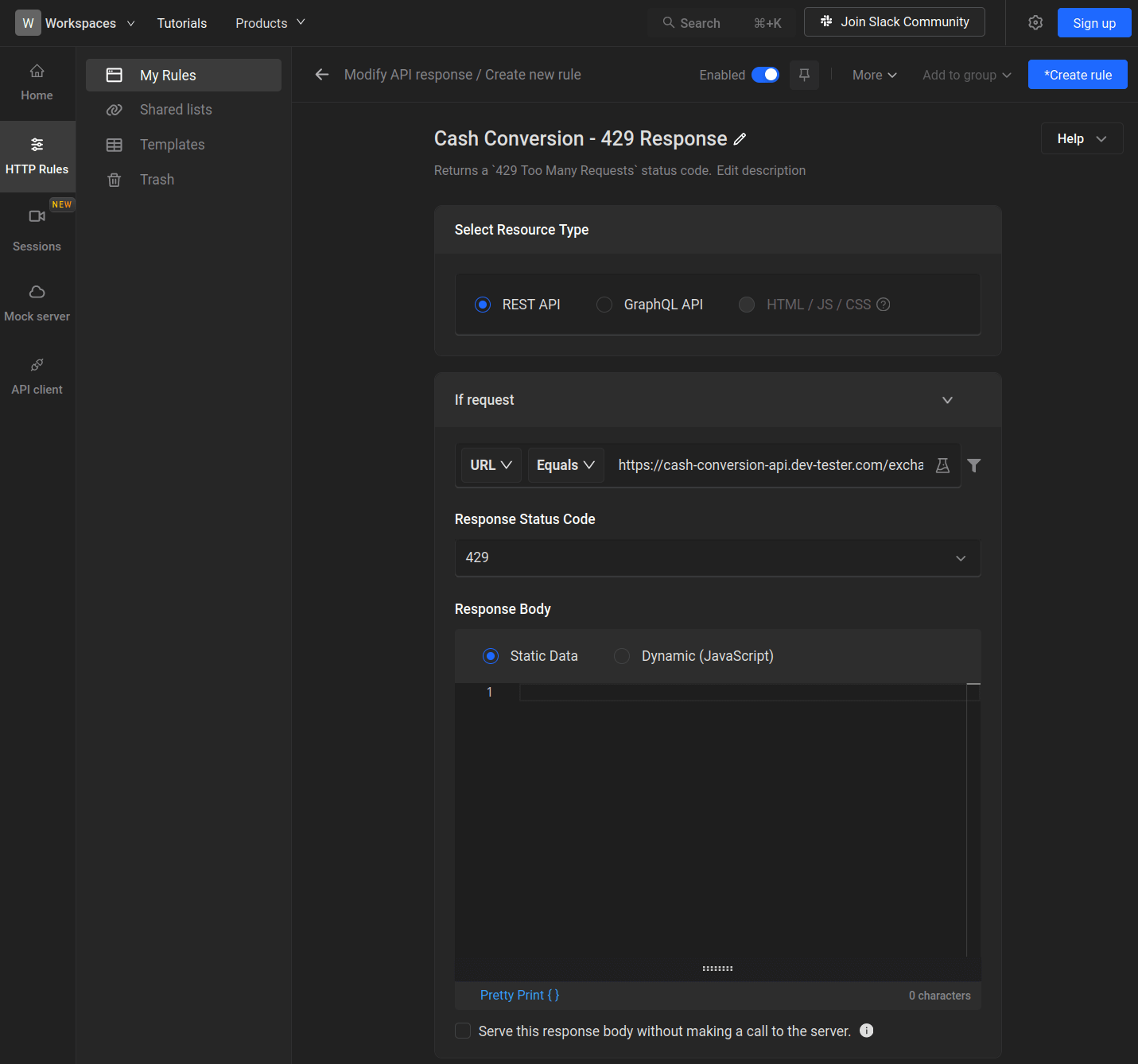
With the rule active, let's try it out on the Cash Conversion app by filling out the form normally and submitting it to trigger the API request. After testing it out, we can see the frontend handles it the same way as when we sent the invalid request earlier. Based on this investigation, we can assume that the frontend will handle all errors similarly. In a real-world application, you might bring this information up to the product stakeholders in case not handling errors on the frontend with different messages is an oversight by development.
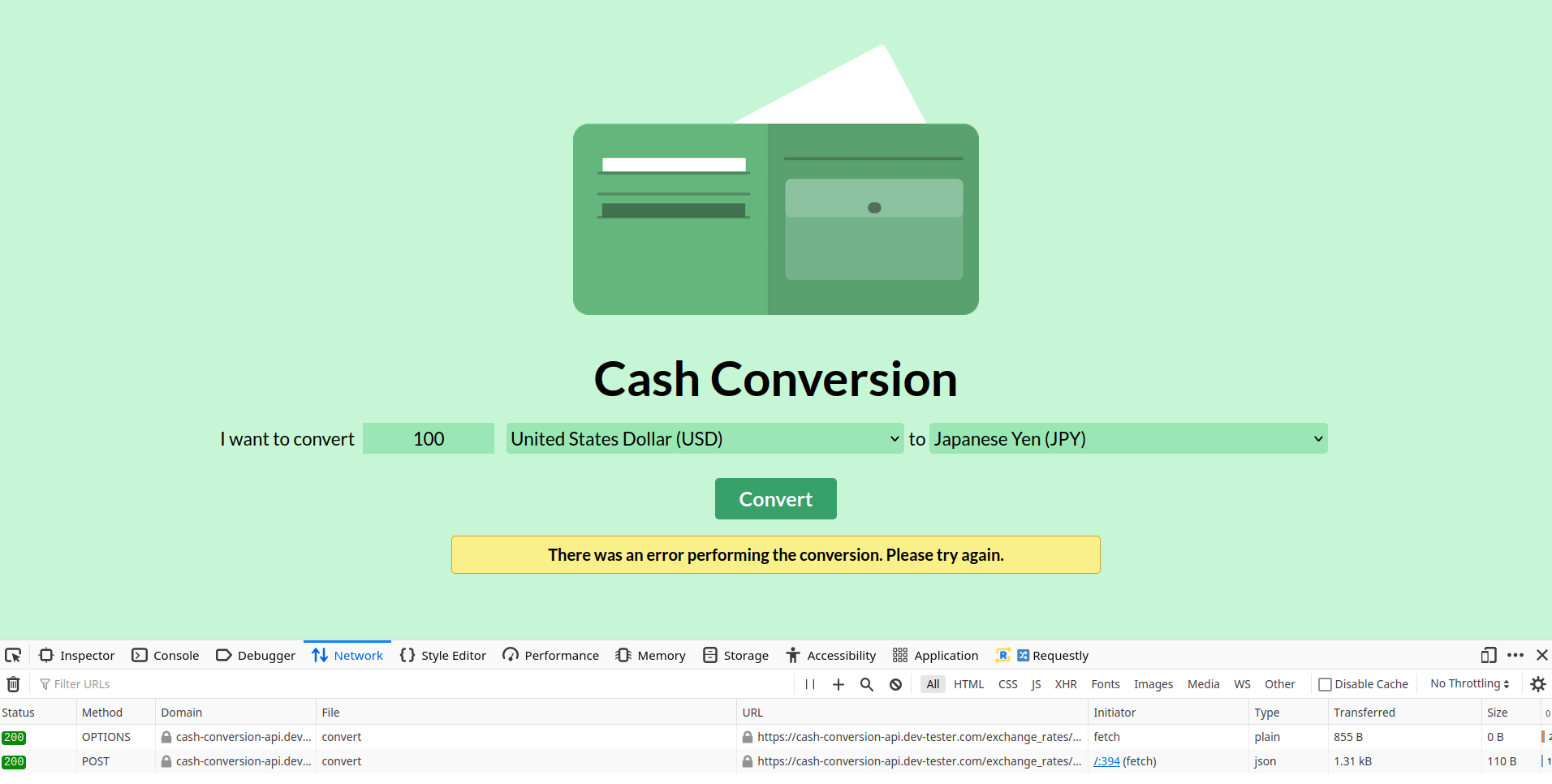
You might observe in the above screenshot that the API responded with a 200 OK
status code, but the frontend still showed an error message. Why is that? One limitation when modifying an API response with Requestly is that your web application will still go through the standard network request and response cycle, but the Requestly extension will change the response after receiving it. When creating the new rule, the extension will display a modal notifying you of this caveat. While the browser still shows the actual response from the backend, the frontend will use the intercepted one by Requestly. This issue might trip you up if you have one of these rules enabled in your browser but forgot about it, which has happened to me a few times already.
Replaying Your Testing Sessions With Requestly
These examples of setting up HTTP rules with the Requestly extension just scratch the surface of how you can change how your web application frontend interacts with the backend without requiring development changes. This article won't go through the other types of rules provided by Requestly. Take some time to explore setting up various rules to better understand how you can use Requestly to speed up your testing.
Beyond setting up rules, however, Requestly contains a lot of other functionality that helps with testing web applications. One of the recent newer features added is Session Replays, a handy tool that can boost any team's quality processes. At a high level, Session Replays allow you to record up to five minutes of your browser during testing sessions and easily share them with your team. However, it's more than just your run-of-the-mill screen capture tool.
Requestly's Session Replays also capture additional information from the recorded session, like the network requests made by the application, the responses from the backend, console messages, and all of your system's details. For testers, you won't have to waste time collecting this data as it's handled automatically for you. Developers will then have access to this valuable information to help them replicate potential bugs and find out where to fix the problem. The amount of time this functionality can save entire teams is significant.
Let's give Session Replays a try on the Cash Conversion app. To start recording a session, click on the Sessions section on the sidebar of the Requestly app. It will show you some information about how the function works. All you need to do is enter the URL you want Requestly to begin recording and click the Start Recording button.
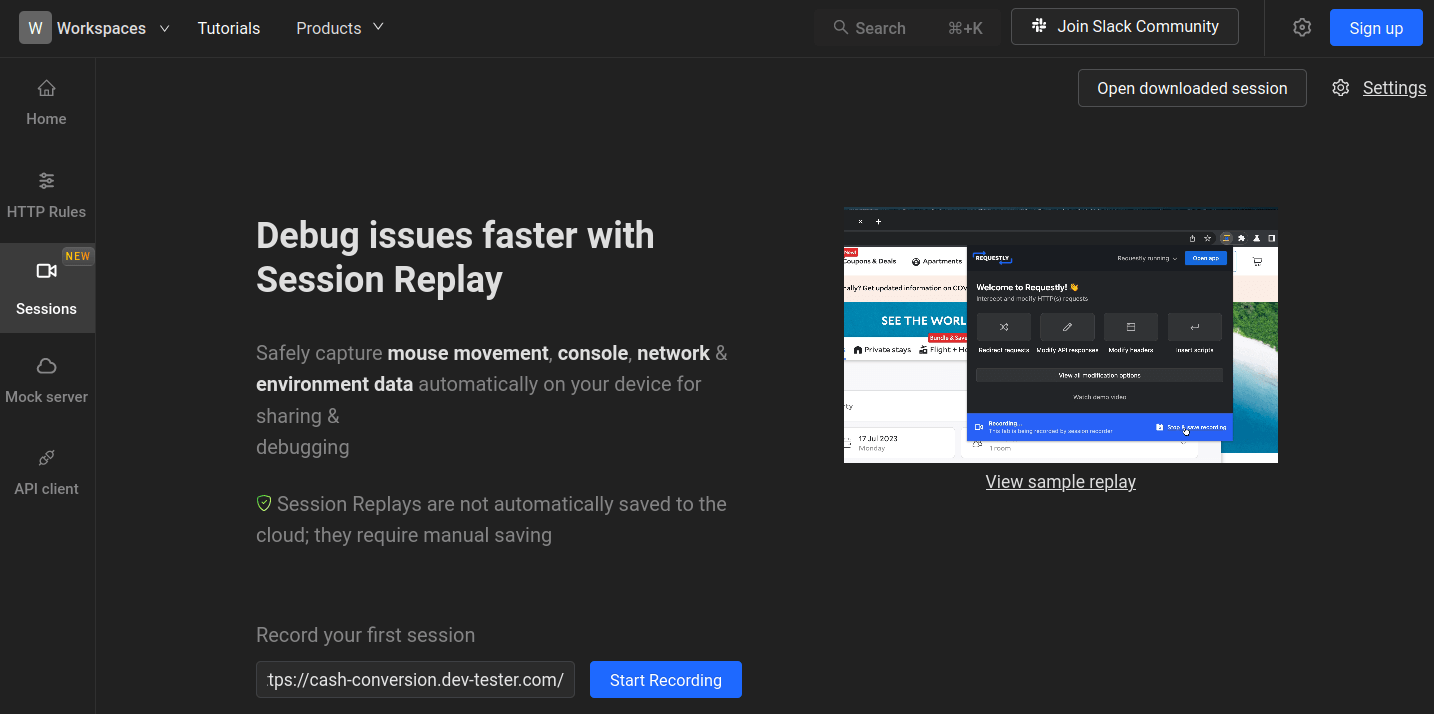
The URL will open in a new tab. You can begin performing your tests by interacting with the application as usual, and Requestly will capture video and additional data from the session.
For this example, let's imagine we're doing exploratory testing on the application, going through some basic scenarios to ensure that the site works as expected and going through untested scenarios. As part of our exploration, we'll check if the frontend or backend can handle negative amounts for conversion. During our testing session, we noticed the application returned the generic error message when attempting to convert a negative amount, so we'll want to flag this as a potential bug.
After wrapping up the testing session, go to the Requestly app or the extension on the browser's address bar to stop the recording. Requestly will open a new window showing the captured session. You'll see a video recording of all you did, along with the network requests and responses. Requestly also tracks your mouse movements, which provides another data point for debugging (the recorded movements showed rather strangely in the example video recording for some reason).
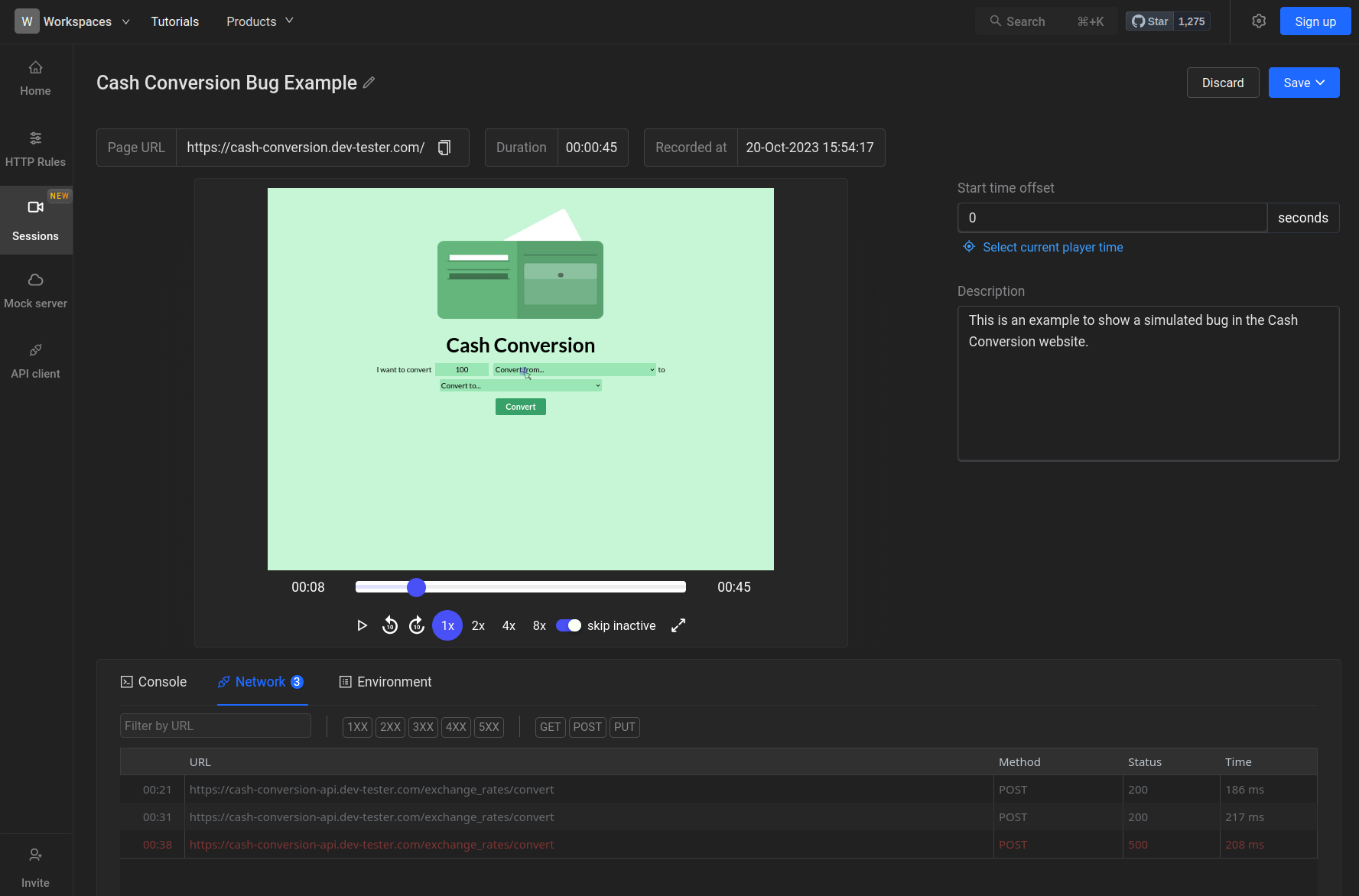
On this screen, you can review the video and other information captured by Requestly. Then, set the session name and description. You can also trim the video by changing its starting time to remove any unnecessary details when starting the recording.
After reviewing the session, you can download the video file on your local system or upload the session to Requestly's servers. You can keep the session private, share it with specific people through email, or generate a link to share it with anyone. To see a real example of this, here's a session I recorded that simulates the imaged scenario mentioned above in the Cash Conversion app.
Summary: Requestly Provides Excellent Value for Testers
By now, you've briefly seen how helpful Requestly can be for developers and testers alike. This article showed a couple of the basics of the tool, but it barely touched on everything you can do using Requestly. Check out their documentation and YouTube page for more details. We didn't get into many of the other HTTP rules or show the mock server or shared lists capabilities, so there's a lot of additional ground to explore, which I might do in future articles—leave a comment below if you're interested in that.
This article isn't sponsored by the company or anyone affiliated with them. But I can say Requestly has been one of the better browser extensions I've used since it contains a ton of functionality in a single place. You can perform many tests right in the browser instead of juggling multiple external tools and services. I've used a few similar tools for testing and debugging purposes throughout my career, and Requestly is one of the best values you can get at this time. I encourage anyone working with web applications to give it a try.
What other tools do you use to explore and test your web applications? Share them with others in the testing community by leaving a comment below!